The WordPress.org plugin repository is filled with coming soon and maintenance mode plugins. My go-to plugin is the one created by SeedProd. However, I often don’t need all of those fancy features and prefer to just do it manually with custom code. Today I’m sharing a few DIY maintenance mode options for web developers.
Option #1: Turn off front-end for visitors only. 🔒
This option works nicely as a coming soon plugin. Visitors will see a custom message whereas logged in users will be able to see the front end. To set this up, create a must-use plugin. This is simply a PHP file, which can be named anything and put inside of wp-content/mu-plugins/
. See the following captaincore_migrate.php
example.
<?php
function captaincore_template_redirect_migrate() {
if ( is_user_logged_in() ) {
return;
}
?><html>
Maintenance Mode Enabled
</html><?php
die();
}
add_action( 'template_redirect', 'captaincore_template_redirect_migrate' );
Option #2: Turn off front-end for everyone. 🔒🔒
This option is a bit more locked down and prevents everyone from seeing the front-end of the website. This is useful for website migrations or a temporary maintenance mode. Start by using the same must-use plugin as shown above. Simply remove the is_user_logged_in()
check and that’s it.
<?php
function captaincore_template_redirect_migrate() {
?><html>
Maintenance Mode Enabled
</html><?php
die();
}
add_action( 'template_redirect', 'captaincore_template_redirect_migrate' );
Option #3: Turn off front-end and back-end for everyone. 🔒🔒🔒
This option is handy for migrating high traffic dynamic websites where you want to make sure nothing is changed. This can be accomplished by creating a custom .maintenance
file at the root of WordPress.
<?php
// Prevents WordPress from ending maintenance mode
$upgrading = time();
?><html>
Maintenance Mode Enabled
</html>
<?php
// Prevents WordPress from loading default message
die();
?>
Be careful. The only way to deactivate this maintenance mode is by manually deleting .maintenance
directly from the web server. If you don’t have SFTP or SSH access, don’t create this file or you’ll lock yourself out.
Customizing maintenance mode pages with regular old HTML and CSS.
The above examples are just to show you how to turn off sections of the website. They’re pretty boring, just a simply message output. Let’s fix that with some HTML and CSS. You can output any arbitrary code, so really there is no limit. Here is a common format I use, a simple message box with a logo image.
<?php
function captaincore_template_redirect_migrate() {
?><html>
<head>
<meta charset="utf-8">
<title>Scheduled Maintenance</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/materialize/1.0.0/css/materialize.min.css">
<link href="https://fonts.googleapis.com/icon?family=Material+Icons" rel="stylesheet">
<style>
@import url('https://fonts.googleapis.com/css?family=Roboto');
body {
font-family: 'Roboto', sans-serif;
}
</style>
</head>
<body class="grey lighten-3">
<div class="container">
<div class="section">
<div class="row">
<div class="col s12 m3"></div>
<div class="col s12 m6">
<div class="card center center-align">
<div class="card-content">
<span class="card-title">Scheduled Maintenance</span>
<p>Will be back shortly.</p>
</div>
<div class="card-content">
<img src="https://anchor.host/wp-content/uploads/2015/01/logo.png" width="170">
</div>
</div>
</div>
<div class="col s12 m3"></div>
</div>
</div>
</div>
</body>
</html><?php
die();
}
add_action( 'template_redirect', 'captaincore_template_redirect_migrate' );
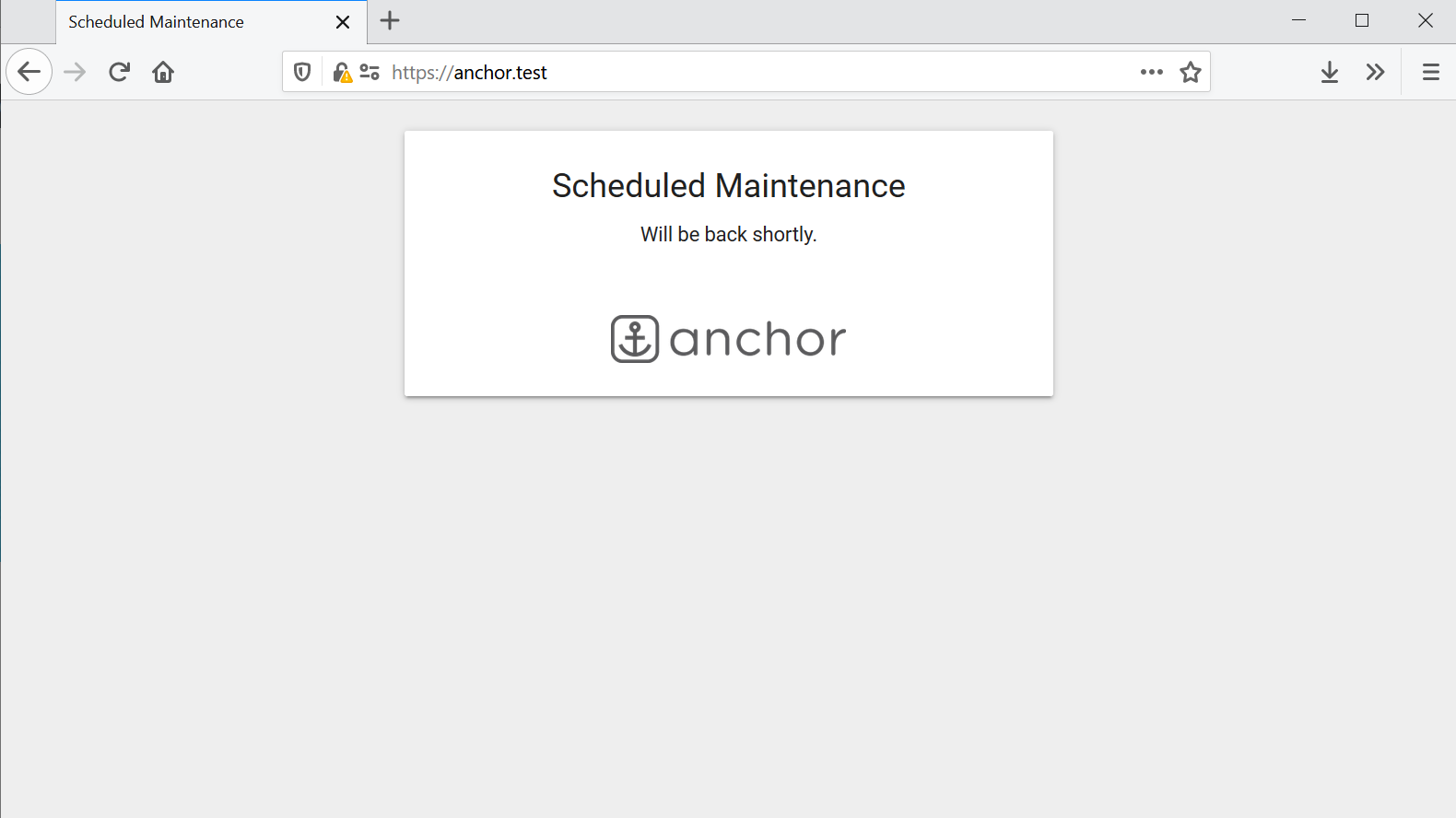