Let’s say you have a list of URLs. What’s the fastest way to scan all websites to see which are using a wildcard SSL? This is a follow-up to a post I wrote earlier this year, You Don’t Need A Wildcard SSL With Kinsta. I’ve been actively replacing Kinsta wildcard SSLs with standard SSLs to avoid yearly manual verification steps. I thought, wouldn’t it be great if I could scan all of my websites and see which ones are still using the wildcard SSL? With a little PHP and bash scripting, that’s just what I did.
Create a file name ssh-wildcard-scanner.sh
with the following. Replace the URLs list with a list of your own. Grant execute permissions chmod +x ssh-wildcard-scanner.sh
and run ./ssh-wildcard-scanner.sh
. Enjoy!
#!/usr/bin/env bash
urls=(
https://anchor.host
https://captaincore.io
https://austinginder.com
)
for url in ${urls[@]}; do
domain=${url/http:\/\/www./} # removes http://www.
domain=${domain/https:\/\/www./} # removes https://www.
domain=${domain/http:\/\//} # removes https://
domain=${domain/https:\/\//} # removes http://
domain=$( echo $domain | awk '{$1=$1};1' ) # Trims whitespace
ssl_response=$( echo | openssl s_client -servername $domain -connect $domain:443 2>/dev/null | openssl x509 -text | grep "DNS:" )
read -r -d '' php_code << heredoc
\$ssl_response = '$ssl_response';
preg_match_all( "/DNS:([a-zA-Z\d\-.*]+)/", \$ssl_response, \$matches );
echo implode( " ", \$matches[1] );
heredoc
domains=$( php -r "$php_code" )
if [[ "$domains" == *"*."* ]]; then
echo "Wildcard SSL found on $url. SSL covers $domains"
else
echo "Standard SSL found on $url. SSL covers $domains"
fi
done
If you are using ZSH or an alternative bash environment then most likely you can just copy and paste the entire code right into a command line without making a script file.
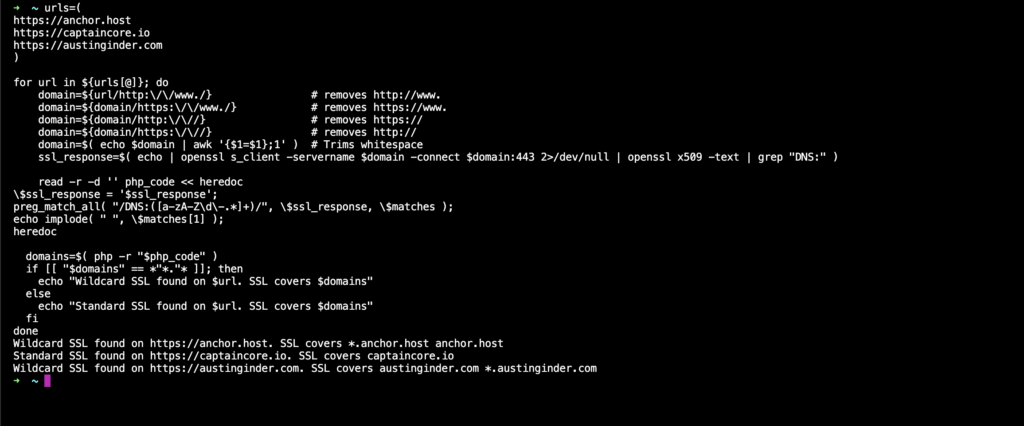